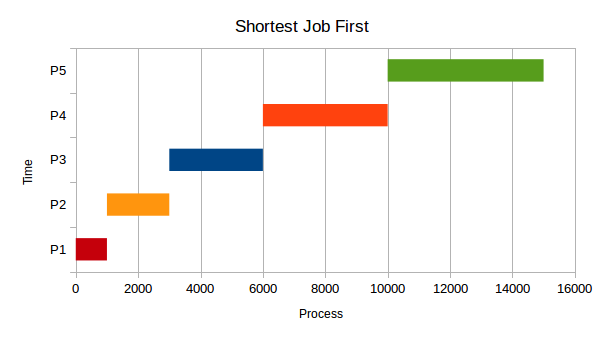
SJF CPU SCHEDULING ALGORITHM WITH C++ PROGRAM
BASIC
SJF stands for Shortest Job First. In this scheduling algorithm there is no effect of arrival time whether they are 0 or not. Shortest scheduling algorithm states that the shortest process is allocated the CPU first. It is implemented by using the queue after shorting the processes according to the burst time. When a process enters the ready queue, its PCB is linked onto the tail of the queue. When the CPU is free, it is allocated to the process at the head of the queue. The running process is then removed from the queue. SJF is a preemptive scheduling algorithm. Non-preemptive SJF turns to SRTF scheduling.
Related Terms
Arrival Time: Time at which the process arrives in the ready queue.
Completion Time: Time at which process completes its execution.
Burst Time: Time required by a process for CPU execution.
Turn Around Time: Time a process needs to be done after arrival.
Waiting Time: Time that a process wait to be executed after arrival.
Turn Around Time = Completion Time – Arrival Time
Completion Time = Turn Around Time + Arrival Time
Turn Around Time = Burst Time + Waiting Time
C PROGRAM
#include<bits/stdc++.h> using namespace std; int main(){ int n, bt[20], wt[20], tat[20], avwt=0, avtat=0, i, j, index[20], itemp, temp; cout<<"Enter Number of Processes: "; cin>>n; cout<<"Enter Process Burst Time:"<<endl; for(i=0;i<n;i++){ cout<<"P["<<i+1<<"]:"; cin>>bt[i]; index[i]=i; } for(i=0;i<n;i++){ for(j=i;j<n;j++){ if(bt[j]<bt[i]){ swap(bt[i], bt[j]); swap(index[i], index[j]); } } } wt[0]=0; cout<<endl<<"Process\t\tBurst Time\tWaiting Time\tTurnaround Time"<<endl; for(i=0;i<n;i++){ wt[i+1]= wt[i]+bt[i]; tat[i]= wt[i]+bt[i]; avwt=avwt+wt[i]; avtat=avtat+tat[i]; cout<<"P["<<index[i]+1<<"]\t\t"<<bt[i]<<"\t\t"<<wt[i]<<"\t\t"<<tat[i]<<endl; } cout<<endl<<"Average Waiting Time: "<<avwt/i<<endl; cout<<"Average Turnaround Time: "<<avtat/n<<endl; return 0; }
OUTPUT
Enter Number of Processes: 10 Enter Process Burst Time: P[1]: 5 P[2]: 7 P[3]: 3 P[4]: 8 P[5]: 12 P[6]: 8 P[7]: 4 P[8]: 9 P[9]: 20 P[10]: 1 Process Burst Time Waiting Time Turnaround Time P[10] 1 0 1 P[3] 3 1 4 P[7] 4 4 8 P[1] 5 8 13 P[2] 7 13 20 P[4] 8 20 28 P[6] 8 28 36 P[8] 9 36 45 P[5] 12 45 57 P[9] 20 57 77 Average Waiting Time: 21 Average Turnaround Time: 29
EXPLANATION
The first line is to including header file and second line is to reserve std namespace.
#include<bits/stdc++.h> using namespace std;
Then we started the main function that is mandatory in C/C++. We declared some variables and array that will be used to store data.
n = Number of Process
bt = Burst Time
wt = Wating Time
tat = Turnaround Time
avwt = Average Waiting Time
avtat = Average Turnaround Time
i = A simple variable to continue loop and define address of array.
j = A simple variable to continue loop and define address of array.
index = An array to store the process number.
itemp = A temporary variable to hold index.
temp = A temporary variable to hold burst time or others.
int main(){ int n, bt[20], wt[20], tat[20], avwt=0, avtat=0, i, j, index[20], itemp, temp;
Ask for the number of process to the user.
cout<<"Enter Number of Processes: "; cin>>n;
As there are more than one process (generally) so we need a loop to get input of burst time of the processes. Ask user for burst time for different process one by one. Save the process number into index array.
cout<<"Enter Process Burst Time:"<<endl; for(i=0;i<n;i++){ cout<<"P["<<i+1<<"]:"; cin>>bt[i]; index[i]=i; }
Sort all processes according to the burst time. ALso sort the index array so that the process number remain same. Here I used bubble sort. You can use any other sorting algorithm also.
for(i=0;i<n;i++){ for(j=i;j<n;j++){ if(bt[j]<bt[i]){ swap(bt[i], bt[j]); swap(index[i], index[j]); } } }
In Shortest Job First Scheduling the first process never wait for any process. So initialize the value of wating time for first process to 0.
wt[0]=0;
Print four column for the process, burst time, waiting time and turnaround time. Below of the corresponding name respective time will be printed.
cout<<endl<<"Process\t\tBurst Time\tWaiting Time\tTurnaround Time"<<endl;
Take a loop for calculating and printing waiting time and turnaround time. Waiting time of a process will be the sum of the waiting time and burst time of previous process. And the turnaround time of a process will be the sum of the waiting time and burst time of that process. avwt and avtat will sum up the total waiting time and total turnaround time in this loop. See the loop below.
for(i=0;i<n;i++){ wt[i+1]= wt[i]+bt[i]; tat[i]= wt[i]+bt[i]; avwt=avwt+wt[i]; avtat=avtat+tat[i]; cout<<"P["<<index[i]+1<<"]\t\t"<<bt[i]<<"\t\t"<<wt[i]<<"\t\t"<<tat[i]<<endl; }
Print out the average waiting time and average turnaround time by dividing the summed time in the loop by n.
cout<<endl<<"Average Waiting Time: "<<avwt/i<<endl; cout<<"Average Turnaround Time: "<<avtat/n<<endl;
Return the main function to 0. End the function with bracket.
return 0; }
I Think Your Experience with this tutorial is quite good. If You have to ask anything comment. Thank You.